by admin
Check Poker Hand Java
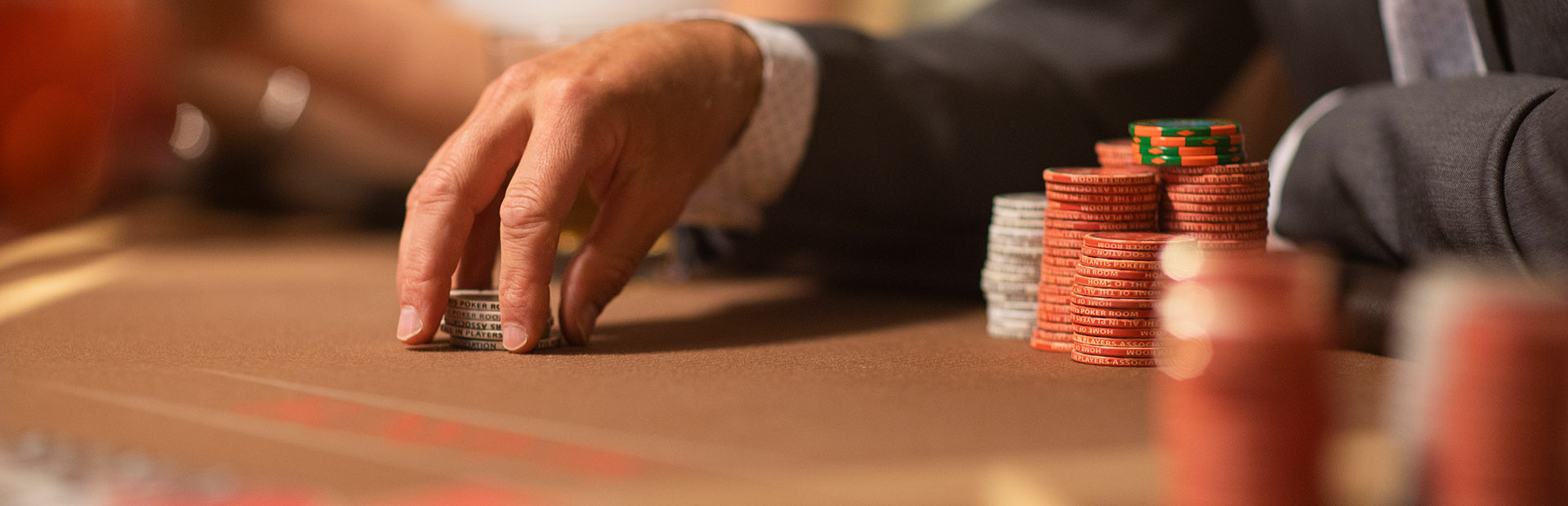
Java Poker Game Code
|

GitHub Gist: instantly share code, notes, and snippets.
Video Poker Java

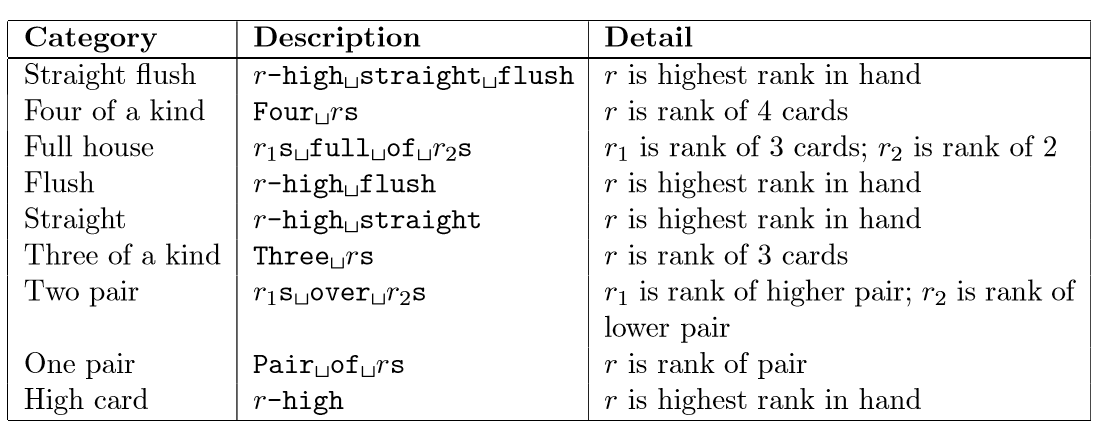
Check Poker Hand Java Download
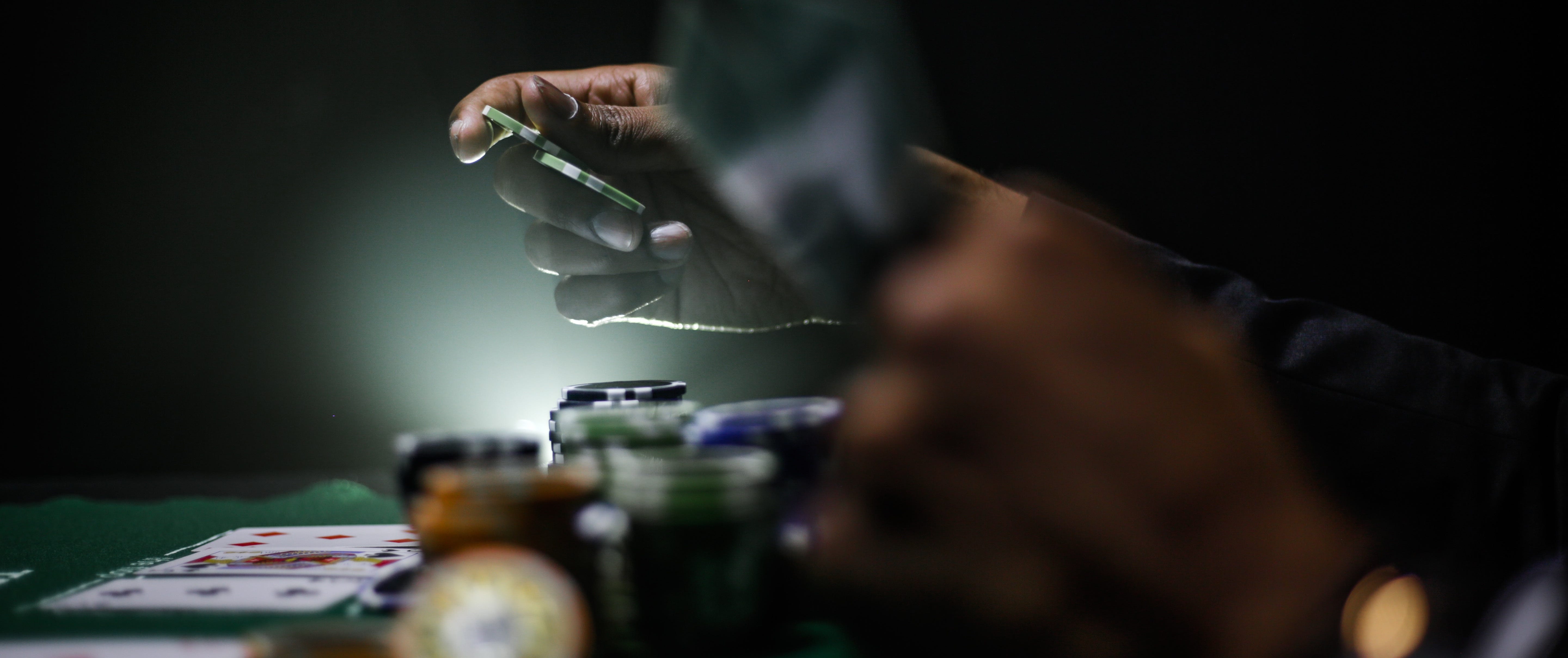
- Poker Hand Frequencies: GitHub Code: https://github.com/amir650/MyCardGame.
- The poker odds calculators on CardPlayer.com let you run any scenario that you see at the poker table, see your odds and outs, and cover the math of winning and losing poker hands. Texas Hold'em Omaha.
- The problem asked me to find the highest possible hand for a given set of cards, so I tried to keep things simple by writing a checkhand function that checks each hand starting from straight flush down to high card. As soon as a condition for a hand was satisfied, I returned a number that corresponded to the strength of the hand (1 for high.
- I'm trying to evaluate a hand to see if they have a pair and I feel like this is right but i keep getting errors. Java - determining is Poker Hand has a Pair.